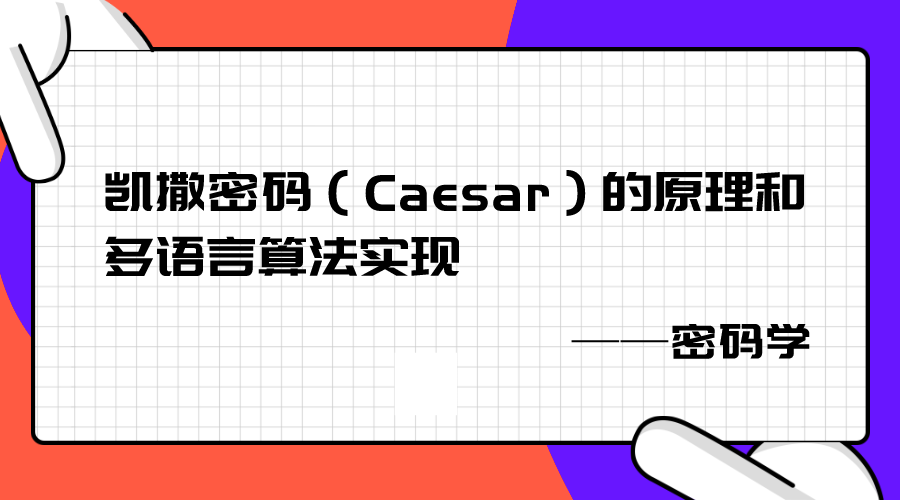
凯撒密码(Caesar)的原理和多语言算法实现
本文最后更新于 2023-11-07,请注意辨别文章的编写时间哦。
1、简介
密码学的历史中有很多经典的加密算法,而凯撒密码(Caesar Cipher)是其中最简单且广为人知的一种。它以古罗马时期的凯撒大帝命名,通过对字母进行固定偏移量的移位操作来加密和解密消息。
本文将介绍凯撒密码的原理,并提供几种常见编程语言的算法实现示例,帮助大家更好地理解和应用凯撒密码。
2、原理
凯撒密码是一种古典密码体质下的一种密码,是一种移位密码,具有单表密码的性质,密文和明文都使用同一个映射,为了保证加密的可逆性,要求映射都是一一对应。
凯撒密码的基本原理是将明文中的每个字母按照一个固定的偏移量向后移动,生成密文。例如,当偏移量为3时,字母A
将被替换为D
,字母B
将被替换为E
,以此类推。解密过程则是将密文中的每个字母按照相同的偏移量逆向移动,还原为明文。
3、公式
- 加密公式:
f(a)=(a+N) mod 26
- 解密公式:
f(a)=(a+(26-N)) mod 26
其中
N
代表的是位移数,也可以算是k
4、代码实现
4.1、C语言版本
C语言
实现的凯撒密码的加解密
代码:
#include <stdio.h>
#include <string.h>
#include <ctype.h>
// 加密
void caesarEncrypt(char *text, char *result, int k) {
int length = strlen(text);
for (int i = 0; i < length; i++) {
char ch = text[i];
if (isalpha(ch)) {
char base;
if (islower(ch)) {
base = 'a';
} else {
base = 'A';
}
result[i] = (ch - base + k) % 26 + base;
} else {
result[i] = ch;
}
}
}
// 解密
void caesarDecrypt(char *text, char *result, int k) {
int length = strlen(text);
for (int i = 0; i < length; i++) {
char ch = text[i];
if (isalpha(ch)) {
char base;
if (islower(ch)) {
base = 'a';
} else {
base = 'A';
}
result[i] = (ch - base - k + 26) % 26 + base;
} else {
result[i] = ch;
}
}
}
int main() {
char text[50];
char result[50];
int k;
int type;
printf("--------欢迎使用凯撒密码-----------\n");
printf("请输入明文或密文:");
scanf("%[^\n]", text);
printf("请选择操作类型:\n");
printf("1. 加密\n");
printf("2. 解密\n");
scanf("%d", &type);
printf("请输入密钥k(正整数):");
scanf("%d", &k);
if (type == 1) {
caesarEncrypt(text, result, k);
printf("加密后的密文:%s\n", result);
} else if (type == 2) {
caesarDecrypt(text, result, k);
printf("解密后的明文:%s\n", result);
} else {
printf("无效的操作类型。\n");
}
return 0;
}
验证:
1、加密验证
2、解密验证
4.2、C++版本
此代码使用了C++
的输入输出流(iostream
)库,以及std::string
类来处理字符串。其他部分与之前的C代码
类似,只是做了一些C++
特定的调整。
代码:
#include <iostream>
#include <string>
#include <cctype>
void caesarEncrypt(std::string& text, std::string& result, int k) {
for (char ch : text) {
if (std::isalpha(ch)) {
char base = std::islower(ch) ? 'a' : 'A';
result += static_cast<char>((ch - base + k) % 26 + base);
} else {
result += ch;
}
}
}
void caesarDecrypt(std::string& text, std::string& result, int k) {
for (char ch : text) {
if (std::isalpha(ch)) {
char base = std::islower(ch) ? 'a' : 'A';
result += static_cast<char>((ch - base - k + 26) % 26 + base);
} else {
result += ch;
}
}
}
int main() {
std::string text;
std::string result;
std::string kString;
int k;
int type;
std::cout << "--------欢迎使用凯撒密码-----------" << std::endl;
std::cout << "请输入明文或密文:";
std::getline(std::cin, text);
std::cout << "请选择操作类型:" << std::endl;
std::cout << "1. 加密" << std::endl;
std::cout << "2. 解密" << std::endl;
std::cin >> type;
std::cout << "请输入密钥k(正整数):";
std::cin >> kString;
try {
k = std::stoi(kString);
} catch (const std::exception& e) {
std::cout << "无效的密钥。" << std::endl;
return 1;
}
std::cin.ignore(); // 忽略之前的换行符
if (type == 1) {
caesarEncrypt(text, result, k);
std::cout << "加密后的密文:" << result << std::endl;
} else if (type == 2) {
caesarDecrypt(text, result, k);
std::cout << "解密后的明文:" << result << std::endl;
} else {
std::cout << "无效的操作类型。" << std::endl;
}
return 0;
}
验证:
1、加密验证
2、解密验证
4.3、Python版本
这个Python
代码使用了字符串操作和条件语句来实现凯撒密码的加密和解密功能。
代码:
def caesar_encrypt(text, k):
result = ""
for ch in text:
if ch.isalpha():
base = ord('a') if ch.islower() else ord('A')
encrypted_ch = chr((ord(ch) - base + k) % 26 + base)
result += encrypted_ch
else:
result += ch
return result
def caesar_decrypt(text, k):
result = ""
for ch in text:
if ch.isalpha():
base = ord('a') if ch.islower() else ord('A')
decrypted_ch = chr((ord(ch) - base - k + 26) % 26 + base)
result += decrypted_ch
else:
result += ch
return result
print("--------欢迎使用凯撒密码-----------")
text = input("请输入明文或密文:")
type = int(input("请选择操作类型:\n1. 加密\n2. 解密\n"))
kString = input("请输入密钥k(正整数):")
try:
k = int(kString)
except ValueError:
print("无效的密钥。")
exit(1)
if type == 1:
encrypted_text = caesar_encrypt(text, k)
print("加密后的密文:" + encrypted_text)
elif type == 2:
decrypted_text = caesar_decrypt(text, k)
print("解密后的明文:" + decrypted_text)
else:
print("无效的操作类型。")
验证:
1、加密验证
2、解密验证
5、扩展和改进
5.1、多偏移量的凯撒密码
在标准的凯撒密码中,每个字母都按照相同的偏移量进行位移操作。然而,我们可以扩展凯撒密码,使得每个字母可以有不同的偏移量。这可以通过使用一个密钥字符串,其中每个字符对应一个字母,并根据该字符的值来确定相应字母的偏移量。
5.2、特殊字符的处理
为了支持特殊字符,我们可以扩展代码来处理这些情况。可以通过将每个字符的ASCII值与字母的基准值进行比较,以确定字符的类型,并根据需要进行位移操作。
5.3、凯撒密码的破解方法和安全性分析
凯撒密码是一种非常简单的替换密码,易于破解。常见的破解方法包括暴力穷举和频率分析。在暴力穷举方法中,尝试所有可能的密钥,并对每个密钥进行解密操作以查看结果是否有意义。频率分析则利用了英文中字母的频率分布特征,通过统计密文中各个字母出现的频率来猜测密钥。
凯撒密码的安全性非常低,容易受到破解。它只有26
个可能的密钥,可以在短时间内被暴力破解。此外,凯撒密码没有考虑语言的语义和上下文,因此容易受到其他更复杂的分析方法的攻击。
6、总结
凯撒密码的主要局限性在于它的密钥空间非常有限,容易受到暴力破解和频率分析等方法的攻击。在现代密码学中,我们需要使用更复杂和安全的加密算法,如对称密钥加密和公钥加密,以提供更高的安全性和保护用户的数据。现代密码学还涉及密钥管理、身份验证、数字签名等方面,以满足安全通信和数据保护的需求。
凯撒密码在历史上具有重要的地位,它为密码学的发展奠定了基础。然而,在实际应用中,我们需要使用更强大和复杂的加密算法来确保信息的安全性。